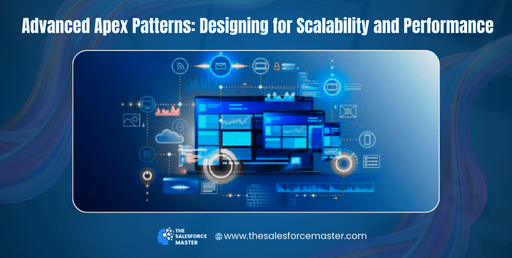
Advanced Apex Patterns: Designing for Scalability and Performance
When working with Salesforce, ensuring that your Apex code is scalable and performs optimally is crucial. Poorly written code can lead to slow response times, governor limit violations, and overall system inefficiencies. Using advanced Apex patterns is key for maintaining high performance in Salesforce solutions, especially when handling large datasets and complex business logic.
1. Use Bulk Patterns for Data Efficiency
Apex code runs in a multi-tenant environment, so adhering to best practices is essential for scalability. Bulk patterns, such as using collections and SOQL for loops, allow your code to handle large volumes of data efficiently. For instance, processing records in batches rather than one by one helps avoid hitting Salesforce governor limits. Salesforce Marketers often face challenges with data volumes, so understanding bulk processing is key.
Here’s an example of using the bulk pattern:
apexCopy codeList<Account> accountsToUpdate = [SELECT Id, Name FROM Account WHERE ...];
for(Account acc : accountsToUpdate) {acc.Name = 'Updated Account';}update accountsToUpdate;
This pattern ensures minimal SOQL queries and DML statements, optimizing performance. When integrated with Salesforce Login systems, seamless handling of authentication data can also benefit from bulk updates, improving user experience.
2. Implement Future, Queueable, and Batchable Apex
One of the most effective ways to ensure your Salesforce platform scales is by using asynchronous processing patterns like Future Methods, Queueable Apex, and Batch Apex. Salesforce limits synchronous processing, so offloading long-running tasks to asynchronous processes ensures that your applications run efficiently without impacting user experience.
- Future Methods allow you to execute operations in the background.
- Queueable Apex provides more complex job chaining and monitoring.
- Batch Apex is suitable for processing large datasets and handling bulk operations.
These patterns are widely adopted by Salesforce Marketers who deal with high data volumes, especially when integrating with marketing platforms like Salesforce Marketing Cloud. By running bulk operations asynchronously, you avoid hitting limits and reduce system stress.
For instance, here’s how Batch Apex is implemented:
apexCopy codeglobal class BatchAccountUpdate implements Database.Batchable<SObject> {global Database.QueryLocator start(Database.BatchableContext bc) {return Database.getQueryLocator('SELECT Id FROM Account WHERE ...');}global void execute(Database.BatchableContext bc, List<SObject> scope) {
=List<Account> accountsToUpdate = (List<Account>) scope;for(Account acc : accountsToUpdate) {acc.Name = 'Updated via Batch';}update accountsToUpdate;}global void finish(Database.BatchableContext bc) {// Optional code to run after batch completes}}
By using Batch Apex, your Salesforce applications can handle massive datasets and ensure the system scales as required.
3. Design Apex for Reusability and Modularity
Another critical component of scalable Apex design is creating reusable and modular code. By following the Separation of Concerns design pattern, you can break your code into smaller, logical pieces. This makes it easier to maintain, test, and scale over time.
A common example of reusability is to create service classes that handle different parts of the business logic. When you modularize your code, it becomes easier to adapt to changes in business needs without modifying large portions of code. This also aligns with Salesforce Marketers who regularly update campaigns or customer segments.
For instance, instead of hardcoding specific logic in triggers, you can offload that logic to a service class:
apexCopy codepublic class AccountService {public static void updateAccountNames(List<Account> accounts) {for(Account acc : accounts) {acc.Name = 'Service Updated';}update accounts;}}
By designing your Apex code in this modular way, you can easily update the logic without disrupting other parts of the system. This is crucial for marketers working with integrated systems, especially when dealing with data from Salesforce Login, ensuring seamless authentication and scalability in user experience.
Conclusion
Using advanced Apex patterns is vital to designing scalable, high-performing applications on Salesforce. By adopting bulk patterns, leveraging asynchronous processing, and following modular design principles, your code will be well-optimized to handle growing business demands. These practices are particularly beneficial for Salesforce Marketers who frequently work with data-intensive applications. Combining these strategies with efficient authentication through Salesforce Login ensures both performance and user satisfaction.
By focusing on these patterns, you will avoid common pitfalls such as governor limit violations, slow performance, and difficult-to-maintain code. Scalable, high-performing solutions are within reach, setting your Salesforce organization up for success in the long run.
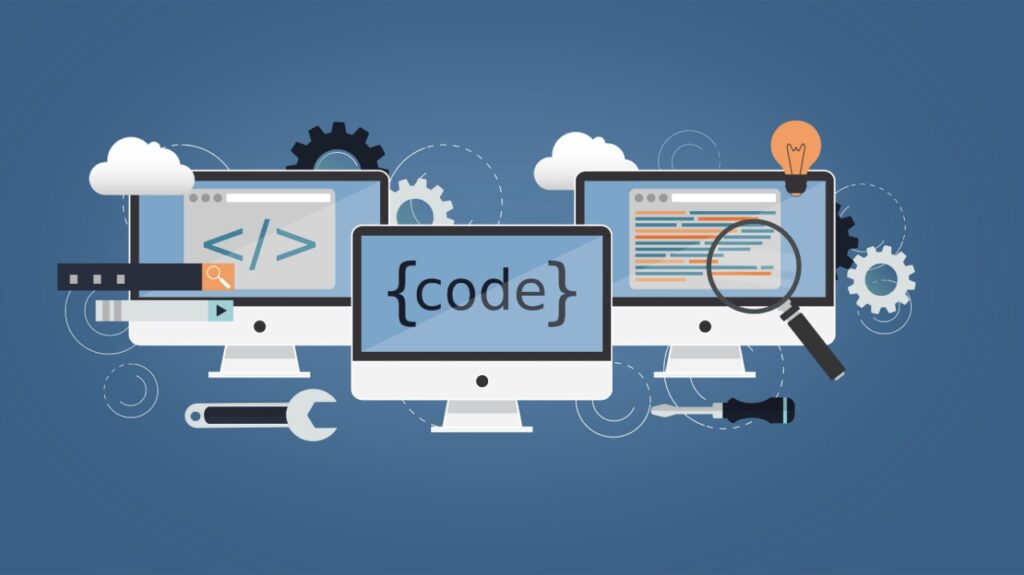